To quickly serve static files in a local development machine, we have used the handy python one-liner to run SimpleHTTPServer
. There is a ruby equivalent to it.
Serve static files
I use this usual goto command, which runs python.
python -m SimpleHTTPServer
There is an equivalent ruby one-liner, which runs WEBrick.
ruby -run -e httpd
This is a pretty powerful server. WEBRick can run a small site/blog without much issues. Read about Richard Schneeman’s experiences here.
Breaking it down
Running ruby --help
gives the various command line options.
The ones in use here are:
-e 'command' one line of script. Several -e's allowed.
-rlibrary require the library before executing your script
By some clever file naming by the ruby devs, there is a library in the ruby source named un.rb
(view source).
This allows to run common unix commands, as this file defines ruby functions for them. For example the following:
ruby -run -e cp -- [OPTION] SOURCE DEST
ruby -run -e touch -- [OPTION] FILE
ruby -run -e mkdir -- [OPTION] DIRS
It also has an httpd
function which runs WEBRick! And it has a list of options as well! You can even configure a ssl certificate if required!
ruby -run -e httpd -- [OPTION] DocumentRoot
--bind-address=ADDR address to bind
--port=NUM listening port number
--max-clients=MAX max number of simultaneous clients
--temp-dir=DIR temporary directory
--do-not-reverse-lookup disable reverse lookup
--request-timeout=SECOND request timeout in seconds
--http-version=VERSION HTTP version
--server-name=NAME name of the server host
--server-software=NAME name and version of the server
--ssl-certificate=CERT The SSL certificate file for the server
--ssl-private-key=KEY The SSL private key file for the server certificate
-v verbose
If you want to modify other options, that WEBrick provides, you can customize as required with a .rb
script.
Here I’m adding a new mime type to the WEBrick server.
require 'webrick'
mime_types = WEBrick::HTTPUtils::DefaultMimeTypes
mime_types.store 'wasm', 'application/wasm'
root = File.expand_path('.')
server = WEBrick::HTTPServer.new(
Port: 8000,
DocumentRoot: root,
MimeTypes: mime_types
)
trap('INT') { server.shutdown } # Ctrl-C to stop
server.start
There are plenty of options. Hack away with WEBrick!
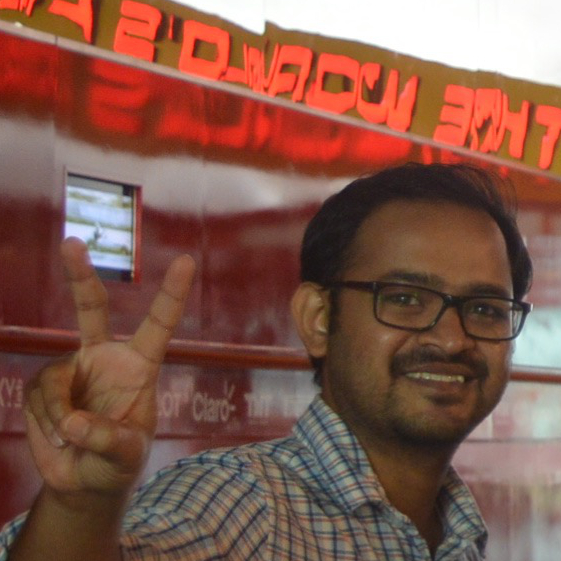